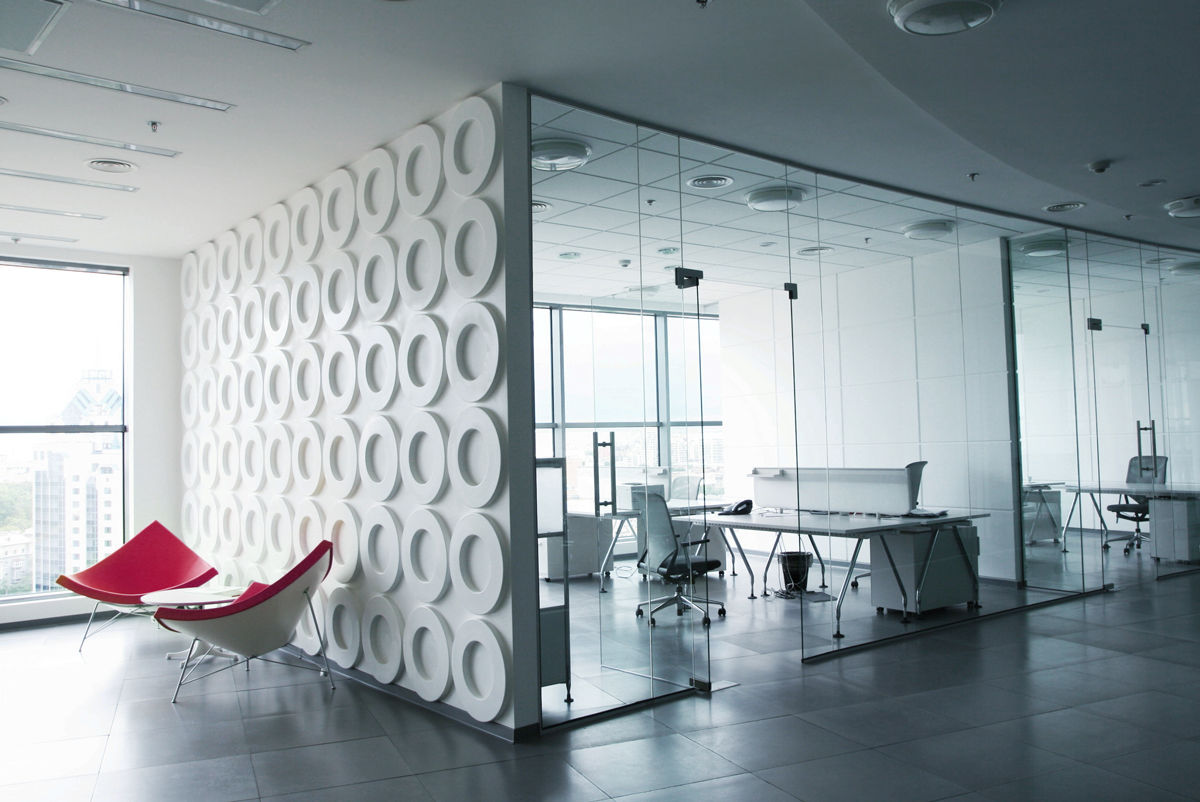
Code exemple :
#include <stdlib.h>
#include <stdio.h>
#include <SDL/SDL.h>
//--------------------------------------------------------------------------------------------
// * Définitions et variables globales *
//--------------------------------------------------------------------------------------------
#define largeur 1280 // fenêtre
#define hauteur 680
#define t_frame 40 // Période timer principal et affichage en ms
SDL_Surface *ecran = NULL; // l'écran
SDL_Surface* bmp = NULL; // image pour blit
SDL_Rect position1; // les rectangles de blit
SDL_Rect position2;
SDL_Rect position3;
SDL_Event event;
SDL_Event user_event;
SDL_TimerID timer; // timer principal
int *paramTimer;
long int Time = 0; // la référence de temps du programme (nombre de période timer principal)
long int clig1 = 0; // Flag de clignotement image
int quitter = 0;
int tempsClic = 0; // temps d'un clic de souris
int tempsMov = 0;
//--------------------------------------------------------------------------------------------
// * Prototypes des Fonctions *
//--------------------------------------------------------------------------------------------
void Initialisation();
void initSDL();
void initPrg();
void finPrg();
void Affichage();
void Actualisation();
void switchEvent();
void gesDown();
void gesClic();
void gesMotion();
void gesKey();
void gesUser();
Uint32 callTimer(Uint32 it, void *para);
//--------------------------------------------------------------------------------------------
// * Fonction MAIN *
//--------------------------------------------------------------------------------------------
int main ( int argc, char** argv )
{
//--------------------------------------------------------------------------------------------
// * INITIALISATION *
//--------------------------------------------------------------------------------------------
Initialisation();
//--------------------------------------------------------------------------------------------
// * BOUCLE PRINCIPALE *
//--------------------------------------------------------------------------------------------
while (!quitter)
{
if (SDL_WaitEvent(&event))
{
switchEvent();
}
Affichage();
}
//--------------------------------------------------------------------------------------------
// * Fin du programme *
//--------------------------------------------------------------------------------------------
// Fin programme
finPrg();
printf("Fin programme OK \n");
return 0;
}
//--------------------------------------------------------------------------------------------
// * FONCTIONS *
//--------------------------------------------------------------------------------------------
void Initialisation()
{
initSDL();
}
void initSDL()
{
// initialize SDL video
if ( SDL_Init( SDL_INIT_VIDEO | SDL_INIT_TIMER) < 0 )
{
printf( "Echec init SDL: %s\n", SDL_GetError() );
}
// make sure SDL cleans up before exit
atexit(SDL_Quit);
// create a new window
ecran = SDL_SetVideoMode(largeur, hauteur, 32, SDL_SWSURFACE|SDL_DOUBLEBUF);
if ( !ecran )
{
printf("Echec ouverture écran video: %s\n", SDL_GetError());
}
// définition d'un User Event
user_event.type=SDL_USEREVENT;
// Lancement du Timer principal
timer = SDL_AddTimer(t_frame, callTimer, ¶mTimer);
// Chargement d'une image
bmp = SDL_LoadBMP("cb.bmp"); // (cb.bmp)
// init position2
position2.x = 150; position2.y = 150;
// init position3
position3.x = 1; position3.y = 1;
}
Uint32 callTimer(Uint32 it, void *para)
{ // Callback du timer principal
// on créé un event pour passer le wait
SDL_PushEvent(&user_event);
return it;
}
void finPrg()
{
SDL_RemoveTimer(timer); // arret timer
SDL_Quit();
}
void switchEvent()
{
switch (event.type)
{
case SDL_QUIT:
quitter = 1;
break;
case SDL_MOUSEBUTTONDOWN:
gesDown();
break;
case SDL_MOUSEBUTTONUP:
gesClic();
break;
case SDL_MOUSEMOTION:
gesMotion();
break;
case SDL_USEREVENT:
gesUser();
break;
case SDL_KEYDOWN:
gesKey();
break;
}
}
void Affichage()
{
// clear ecran
SDL_FillRect(ecran, 0, SDL_MapRGB(ecran->format, 0, 0, 0));
// draw bitmap l'image1 qui suit la souris
SDL_BlitSurface(bmp, 0, ecran, &position1);
// l'image2 qui clignote toute seule
if(clig1) SDL_BlitSurface(bmp, 0, ecran, &position2);
// l'image3 qui se déplace dans l'écran
SDL_BlitSurface(bmp, 0, ecran, &position3);
SDL_Flip(ecran);
printf("Instant fin d'affichage (ms) : %i \n",SDL_GetTicks());
}
void gesDown()
{
tempsClic=SDL_GetTicks();
// Instant ButtonDown
printf("clic %i \n",SDL_GetTicks() );
}
void gesClic()
{
tempsClic=SDL_GetTicks()-tempsClic;
// Durée d'un clic :
printf("temps Clic %i \n", tempsClic);
}
void gesMotion()
{
int x = event.button.x;
int y = event.button.y;
// la position de la souris
printf("x = %i \n", x);
// l'image suit la souris
position1.x = x; position1.y = y;
tempsMov=SDL_GetTicks();
// l'instant de positionnement
printf("motion %i \n", tempsMov);
}
void gesUser()
{
// On incrémente notre référence de temps
Time++;
// Actualisation pour image2
clig1=Time/15 %2;
// Actualisation pour image3
// Vecteur vitesse
static int vx=4;
static int vy=4;
static int dirx=1;
static int diry=1;
// Les positions évoluent en fonction de la vitesse
position3.x = position3.x + dirx*vx;
position3.y = position3.y + diry*vy;
// On change de sens sur les bords
if(position3.x>largeur) dirx = -1;
if(position3.y>hauteur) diry = -1;
if(position3.x<0) dirx = 1;
if(position3.y<0) diry = 1;
}
void gesKey()
{
}
Retour SOMMAIRE